- Poker Checker 1.0.1 By Ddm 2
- Poker Checker 1.0.1 By Ddm 1
- Poker Checker 1.0.1 By Ddm 1
- Poker Checker 1.0.1 By Ddm 5
- Poker Checker 1.0.1 By Ddm 3
IGG.COM, the developer behind Texas HoldEm Poker Deluxe and Castle Clash has released Nova Force for Android. This is an Arcade game that lets you create your very own starcrafts, and amass the most powerful galactic force and smash the miscreants into space debris.
This first post will show a poker game class in Java that was created for a computer science class. I want to further expand and cleanup this code to make it fully understandable, while giving a good tutorial on Java programming.code:
import java.util.Scanner;
/**
* Program: Poker
*
* Description:
* This is the class that contains computer ai, the rules of poker, and the logic
* for creating a deck of cards.
*
* @author Michael S.
*/
public final class Poker {
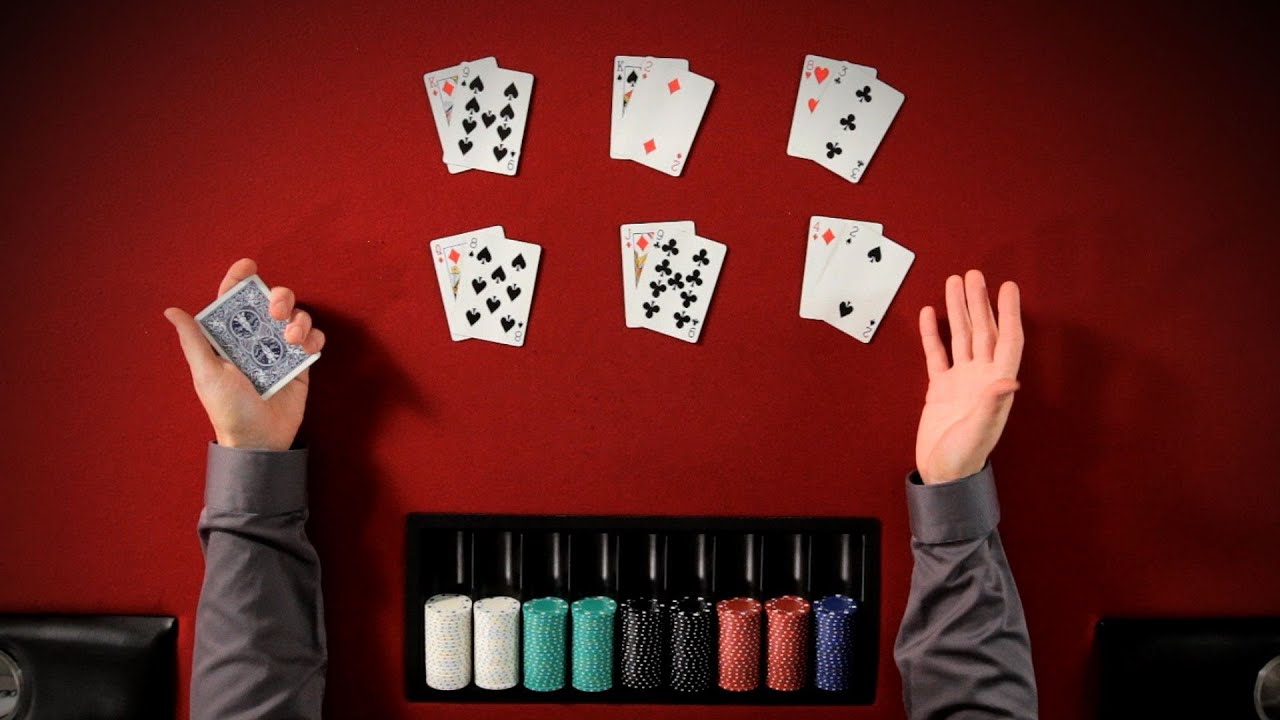
String[] suits = {'Hearts', 'Diamonds', 'Spades', 'Clubs'};
char[] value = {'2', '3', '4', '5', '6', '7', '8', '9', 'T', 'J', 'Q', 'K', 'A'};
// check cards in deck
char[][] cards = new char[13][4];
// array for [player][card][suit/val]
int[][][] pc = new int[2][5][2];
// comp and players hand, determines winner
int[] hand = new int[2];
int[] handVal = new int[2];
// for ai to complete without hand exposed
boolean aiDone = false;
// user input
Scanner input = new Scanner(System.in);
// for betting
int money = 100;
int bet = 0;
public void clear() {
System.out.println('nn');
}// end of clear
/**
* Make a bet
*/
public void makeBet() {
// check if money is available
if (money <= 0) {
System.out.println('You have no money!!!');
} else {
do {
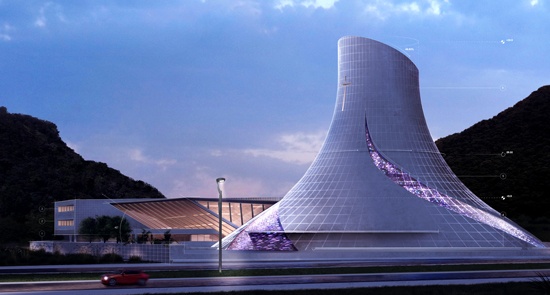
+ '.nPlease place a bet:');
bet = input.nextInt();// user placed bet
} while (!valid(bet, money, 0));
}// end of if else
}// end of makeBet
/**
* Make sure bounds are kept
* @param tester number to be tested
* @param high highest limit
* @param low lowest limit
* @return go or nogo
*/
public boolean valid(int tester, int high, int low) {
if (low <= tester && tester <= high) {
return true; // valid
}// end of if
// not valid
System.out.println('Please enter a valid response');
return false;
}// end of valid
/**
* Generate a hand
* @param player comp or human
*/
public void generateHand(int player) {
// is card taken
boolean cardTaken;
// card
int crd;
// current value
int curVal;
// current suit
int curSuit;
cardTaken = true;
// issue card
for (crd = 0; crd < 4; crd++) {
do {
// create a random value
pc[player][crd][0] = ((int) (Math.random() * 52) % 13);
// create a random suit
pc[player][crd][1] = ((int) (Math.random() * 52) % 4);
curVal = pc[player][crd][0];
curSuit = pc[player][crd][1];
if (cards[curVal][curSuit] 'T') {
cardTaken = true;
} else {
cardTaken = false;
}
} while (cardTaken || pc[player][crd][0] > 12);
cards[curVal][curSuit] = ' ';
}// end of for loop
}// end of generate hand
/**
* Determine type of hand
* @param player comp or human
*/
public void determineHand(int player) {
boolean group1 = false;
// sort cards in numeric order
sortCards(player);
// easy to determine hand
int cardVal1 = pc[player][0][0];
int cardVal2 = pc[player][1][0];
int cardVal3 = pc[player][2][0];
int cardVal4 = pc[player][3][0];
int cardVal5 = pc[player][4][0];
int suitVal1 = pc[player][0][1];
int suitVal2 = pc[player][1][1];
int suitVal3 = pc[player][2][1];
int suitVal4 = pc[player][3][1];
int suitVal5 = pc[player][4][1];
hand[player] = 0;
// determine a pair
if (cardVal1 cardVal2 || cardVal2 cardVal3 || cardVal3 cardVal4
|| cardVal4 cardVal5) {
group1 = true;
hand[player] = 1;
// determine two pair
}
if (group1) {
if (cardVal1 cardVal2 && cardVal3 cardVal4
|| cardVal1 cardVal2 && cardVal4 cardVal5
|| cardVal2 cardVal3 && cardVal4 cardVal5) {
hand[player] = 2;
}
//Three of a kind
if (cardVal1 cardVal2 && cardVal1 cardVal3
|| cardVal2 cardVal3 && cardVal2 cardVal4
|| cardVal3 cardVal4 && cardVal3 cardVal5) {
hand[player] = 3;
}
// determine a fullhouse
if (cardVal1 cardVal2 && cardVal3 cardVal4
&& cardVal3 cardVal5 || cardVal1 cardVal2
&& cardVal1 cardVal3 && cardVal4 cardVal5) {
hand[player] = 6;
}
// determine four of a kind
if (cardVal1 cardVal2 && cardVal1 cardVal3
&& cardVal1 cardVal4 || cardVal2 cardVal3
&& cardVal2 cardVal4 && cardVal2 cardVal5) {
hand[player] = 7;
}
group1 = false;
}
// check suits
Poker Checker 1.0.1 By Ddm 2
if (!group1) {// determine a straight
if (cardVal1 (cardVal2 - 1)
&& cardVal2 (cardVal3 - 1) && cardVal3
(cardVal4 - 1) && cardVal4
(cardVal5 - 1)) {
hand[player] = 4;
}
// determine straight flush
if (suitVal1 suitVal2 && suitVal1 suitVal3
&& suitVal1 suitVal4
&& suitVal1 suitVal5) {
hand[player] = 8;
}
// determine a royal flush
if (cardVal5 14)//Royal Flush
{
hand[player] = 9;
}// end of royalflush if
// determine flush
else if (suitVal1 suitVal2
&& suitVal1 suitVal3 && suitVal1
suitVal4 && suitVal1 suitVal5) {
hand[player] = 5;
} else {// determine just high Card
hand[player] = 0;
}// end of else
}
// display what player has
switch (player) {
case 0:
System.out.println('PLAYER has ');
break;
case 1:
System.out.println('CPU has ');
break;
}
switch (hand[player]) {
case 0:
System.out.println('A HIGH CARD');
break;
case 1:
System.out.println('A PAIR');
break;
case 2:
System.out.println('TWO PAIR');
break;
case 3:
System.out.println('THREE OF A KIND');
break;
case 4:
System.out.println('A STRAIGHT');
break;
case 5:
System.out.println('A FLUSH');
break;
case 6:
System.out.println('A FULL HOUSE');
break;
case 7:
System.out.println('FOUR OF A KIND');
break;
case 8:
System.out.println('STRAIGHT FLUSH');
break;
case 9:
System.out.println('ROYAL FLUSH');
break;
}// end of switch hand
Poker Checker 1.0.1 By Ddm 1
}// end of determine hand
/**
* Determine the string of card value
* @param val index of array
* @return The string value (ie. Ace, 2, 3, ...)
*/
public String cardValString(int val) {
String tmpValStr = ';
if (val 0) {
tmpValStr = '2';
}
if (val 1) {
tmpValStr = '3';
}
if (val 2) {
tmpValStr = '4';
}
if (val 3) {
tmpValStr = '5';
}
if (val 4) {
tmpValStr = '6';
}
if (val 5) {
tmpValStr = '7';
}
if (val 6) {
tmpValStr = '8';
}
if (val 7) {
tmpValStr = '9';
}
if (val 8) {
tmpValStr = '10';
}
if (val 9) {
tmpValStr = 'JACK';
}
if (val 10) {
tmpValStr = 'QUEEN';
}
if (val 11) {
tmpValStr = 'KING';
}
if (val 12) {
tmpValStr = 'ACE';
}
return tmpValStr + ' of ';
}// end of cardValStr
/**
* Determine display string suit
* @param suitVal array index to be converted
* @return suit (ie. hearts, spades,...)
*/
public String suitValStr(int suitVal) {
String tmpSuitStr = ';
if (suitVal 0) {
tmpSuitStr = 'Hearts';
}
if (suitVal 1) {
tmpSuitStr = 'Diamonds';
}
if (suitVal 2) {
tmpSuitStr = 'Spades';
}
if (suitVal 3) {
tmpSuitStr = 'Clubs';
}
return tmpSuitStr;
}// end of suitValStr
/**
* Used for displaying cards
* @param player comp or human
*/
public void cardBuilder(int player) {
int cardVal1 = pc[player][0][0];
int suitVal1 = pc[player][0][1];
int cardVal2 = pc[player][1][0];
int suitVal2 = pc[player][1][1];
int cardVal3 = pc[player][2][0];
int suitVal3 = pc[player][2][1];
int cardVal4 = pc[player][3][0];
int suitVal4 = pc[player][3][1];
int cardVal5 = pc[player][4][0];
int suitVal5 = pc[player][4][1];
// Print the card number
System.out.println('Card 1: ' + cardValString(cardVal1)
+ suitValStr(suitVal1) + 'nCard 2: '
+ cardValString(cardVal2) + suitValStr(suitVal2)
+ 'nCard 3: ' + cardValString(cardVal3)
+ suitValStr(suitVal3) + 'nCard 4: '
+ cardValString(cardVal4) + suitValStr(suitVal4)
+ 'nCard 5: ' + cardValString(cardVal5) + suitValStr(suitVal5));
}// end of cardBuilder
/**
* Computers brain
*/
public void artificialInteli() {
int cardVal1 = pc[1][0][0];
int suitVal1 = pc[1][0][1];
int cardVal2 = pc[1][1][0];
int suitVal2 = pc[1][1][1];
int cardVal3 = pc[1][2][0];
int suitVal3 = pc[1][2][1];
int cardVal4 = pc[1][3][0];
int suitVal4 = pc[1][3][1];
int cardVal5 = pc[1][4][0];
int suitVal5 = pc[1][4][1];
// put hands in order
sortCards(1);
// try a flush
if (hand[1] 0) {
if (hand[1] 0 && suitVal1 suitVal2 && suitVal1 suitVal3
&& suitVal1 suitVal4) {
swapGenerator(1, 4);
} else if (hand[1] 0 && suitVal1 suitVal2
&& suitVal1 suitVal3 && suitVal1 suitVal5) {
swapGenerator(1, 3);
} else if (hand[1] 0 && suitVal1 suitVal2
&& suitVal1 suitVal4 && suitVal1 suitVal5) {
swapGenerator(1, 2);
} else if (hand[1] 0 && suitVal1 suitVal3
&& suitVal1 suitVal4 && suitVal1 suitVal5) {
swapGenerator(1, 1);
} else if (hand[1] 0 && suitVal2 suitVal3
&& suitVal2 suitVal4 && suitVal2 suitVal5) {
swapGenerator(1, 0);
// try straight
} else if (hand[1] 0 && cardVal5 cardVal4 + 1
&& cardVal4 cardVal3 + 1 && cardVal3 cardVal2 + 1) {
swapGenerator(1, 0);
} else if (hand[1] 0 && cardVal4 cardVal3 + 1
&& cardVal3 cardVal2 + 1 && cardVal2 cardVal1 + 1) {
swapGenerator(1, 4);
// keep High card
} else if (hand[1] 0 && handVal[1] >= 10) {
swapGenerator(1, 3);
swapGenerator(1, 2);
swapGenerator(1, 1);
swapGenerator(1, 0);
} else if (hand[1] 0) {
generateHand(1);
}// end of else ifs
}// end of if
// keep a pair
if (hand[1] 1) {
if (cardVal1 cardVal2) {
swapGenerator(1, 2);
swapGenerator(1, 3);
}// end of if
if (cardVal2 cardVal3) {
swapGenerator(1, 0);
swapGenerator(1, 3);
}// end of if
if (cardVal3 cardVal4) {
swapGenerator(1, 0);
swapGenerator(1, 1);
}// end of if
if (cardVal4 cardVal5) {
swapGenerator(1, 0);
swapGenerator(1, 1);
swapGenerator(1, 2);
}// end of if
}// end of keep pair if
Poker Checker 1.0.1 By Ddm 1
// keep two pairif (hand[1] 2) {
if (cardVal1 cardVal2 && cardVal3 cardVal4) {
swapGenerator(1, 4);
}// end of inner if
if (cardVal2 cardVal3 && cardVal4 cardVal5) {
swapGenerator(1, 0);
}// end of inner if
}// end of keep two pair if
// keep three of a kind
if (hand[1] 3) {
if (cardVal1 cardVal2 && cardVal1 cardVal3) {
swapGenerator(1, 3);
swapGenerator(1, 4);
}// end of if
if (cardVal2 cardVal3 && cardVal2 cardVal4) {
swapGenerator(1, 0);
swapGenerator(1, 4);
}// end of if
if (cardVal3 cardVal4 && cardVal3 cardVal5) {
swapGenerator(1, 0);
swapGenerator(1, 1);
}// end of if
}// end of three of a kind if
}// end of ai
/**
* Takes a card from the deck that has not been taken
* @param player computer or human
* @param cNum number of cards
*/
public void swapGenerator(int player, int cNum) {
boolean cardTaken;
int CurVal;
int CurSuit;
do {
cardTaken = true;
pc[player][cNum][0] = ((int) (Math.random() * 52) % 13);
pc[player][cNum][1] = ((int) (Math.random() * 52) % 4);
CurVal = pc[player][cNum][0];
CurSuit = pc[player][cNum][1];
if (cards[CurVal][CurSuit] '8') {
cardTaken = true;
} else {
cardTaken = false;
}
} while (pc[player][cNum][0] > 12);
//cards[CurVal][CurSuit] = '8';
}// end of swapGenerator
/**
* Replace card
*/
public void replaceCard() {
String reply;
boolean rValid;
int num, crd, cardNum;
boolean[] swapped = {true, false, false, false, false, false};
rValid = false;
while (!rValid) {
System.out.print('Do you wish to replace any of your cards?'
+ 'nY(y) or N(n)');
reply = input.next();
if (reply.toUpperCase().equals('Y')) {
System.out.print('How many cards do you want to replace?');
num = input.nextInt();
// replace all cards
if (num 5) {
generateHand(0);
} else {
do {
System.out.print('nWhat card numbers do you want '
+ 'replaced? ');
cardNum = input.nextInt();
} while (!valid(cardNum, 5, 1) && swapped[cardNum] false);
swapped[cardNum] = true;
// cardNum - 1 is used to account for zero based array
swapGenerator(0, cardNum - 1);
for (crd = 1; crd < num; crd++) {
do {
System.out.println(' and ');
System.out.println(');
} while (!valid(cardNum, 5, 1) && swapped[cardNum] false);
swapped[cardNum] = true;
swapGenerator(0, cardNum - 1);
}
}
rValid = true;
} else if (reply.toUpperCase().equals('N')) {
rValid = true;
} else {
System.out.println('Illegal reply. Try again.');
rValid = false;
}
}
sortCards(0);
System.out.print('Press return to continue.');
clear();
System.out.println('PLAYER's cards');
cardBuilder(0);
}
/**
* Sort the array from low to high
* @param player human or computer
*/
public void sortCards(int player) {
// temp value and temp suit
int vTemp, sTemp;
// card
int crd;
int cardVal1 = pc[player][0][0];
int cardVal2 = pc[player][1][0];
int cardVal3 = pc[player][2][0];
int cardVal4 = pc[player][3][0];
int cardVal5 = pc[player][4][0];
int suitVal1 = pc[player][0][1];
int suitVal2 = pc[player][1][1];
int suitVal3 = pc[player][2][1];
int suitVal4 = pc[player][3][1];
int suitVal5 = pc[player][4][1];
for (crd = 0; crd < 4; crd++) {
if (cardVal1 > cardVal2) {
vTemp = cardVal1;
cardVal1 = cardVal2;
cardVal2 = vTemp;
sTemp = suitVal1;
suitVal1 = suitVal2;
suitVal2 = sTemp;
}
if (cardVal2 > cardVal3) {
vTemp = cardVal2;
cardVal2 = cardVal3;
cardVal3 = vTemp;
sTemp = suitVal2;
Poker Checker 1.0.1 By Ddm 5
suitVal2 = suitVal3;
suitVal3 = sTemp;
}
if (cardVal3 > cardVal4) {
vTemp = cardVal3;
cardVal3 = cardVal4;
cardVal4 = vTemp;
sTemp = suitVal3;
suitVal3 = suitVal4;
suitVal4 = sTemp;
}
if (cardVal4 > cardVal5) {
vTemp = cardVal4;
cardVal4 = cardVal5;
cardVal5 = vTemp;
sTemp = suitVal4;
suitVal4 = suitVal5;
suitVal5 = sTemp;
}
}
pc[player][0][0] = cardVal1;
pc[player][1][0] = cardVal2;
pc[player][2][0] = cardVal3;
pc[player][3][0] = cardVal4;
pc[player][4][0] = cardVal5;
pc[player][0][1] = suitVal1;
pc[player][1][1] = suitVal2;
pc[player][2][1] = suitVal3;
pc[player][3][1] = suitVal4;
pc[player][4][1] = suitVal5;
}// end of sortCards
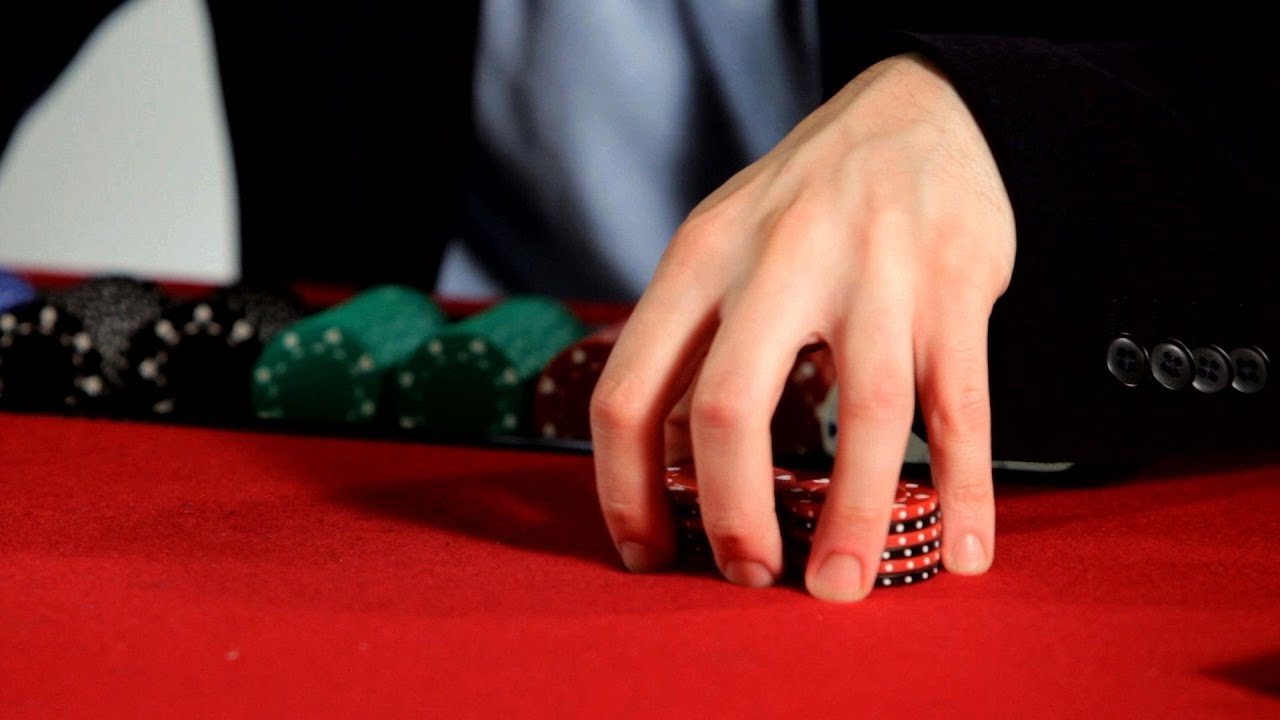
Poker Checker 1.0.1 By Ddm 3
if (hand[0] > hand[1]) {
System.out.println('Player wins');
money = money + bet;
} else {
System.out.println('Computer wins');
money = money - bet;
}
}// end of determine winner
}// end of Poker
note:
- comments in the code for readability for inexperienced programmers(This is good practice)
- variable names should be self explanatory
- to run this program a main method is needed(will show)
The next post will focus on creating separate classes using the above code that makes the program more readable.